Building a Reddit bot with PRAW
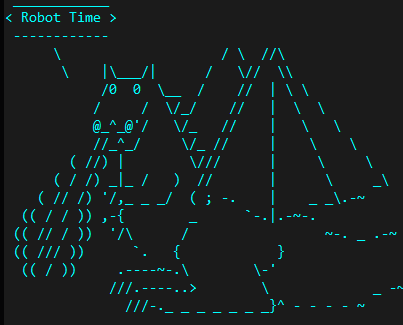
PRAW (Python Reddit API Wrapper) is, as the name suggests, a Python Wrapper for managing API access to Reddit. PRAW takes control over the handling of all API calls to abide by Reddit's rules, so you don't need to worry about it.
Dependency-wise, I am using Python 2.7 here (for familiarity) on a Ubuntu 17.10 machine, and I will be using Python's PIP to install the PRAW packages.
skylar@SkyeNetDev ✗ apt install python
skylar@SkyeNetDev ✗ python --version
Python 2.7.12
skylar@SkyeNetDev ✗ pip install praw
Once we have PRAW installed, we want to populate the Initialization file with our Reddit bot's details, but first we will need to generate our API credentials. Follow the following link:
https://www.reddit.com/prefs/apps/
On the "Create App" dropdown, select "Script" and follow the prompts. You will be provided with a Client_ID and a Secret, which you will need to note down for future use.
Now that you have your API, you will want to fill in the praw.ini
config-file for your praw install. You may find this in your Python install's /usr/local/lib/
dist-packages. At the bottom of the file, add in a section similar to the following and populate accordingly:
[reddit bot]
client_id=
client_secret=
username=
password=
Now we have praw set-up, we can import into our Python script to verify functionality. Try a simple content browser such as the following:
#!/usr/bin/python
import praw
reddit = praw.Reddit('reddit bot')
subreddit = reddit.subreddit("All")
for submission in subreddit.hot(limit=10):
print("Title: ", submission.title)
print("Content: ", submission.selftext)
If all is successful, you should see the Top 10 posts of r/All in your console. If you don't, you may need to exert some Google-Fu.
Now we have API access to read Reddit, we can test some other tools that PRAW makes available to us:
#!/usr/bin/python
import praw
import time
import random
reddit = praw.Reddit('reddit bot')
subreddit = reddit.subreddit("r/All")
comments = subreddit.stream.comments()
AVAILABLE_RESPONSES = ['REPLY1', 'REPLY2', 'REPLY3', 'REPLY4', 'REPLY5']
for comment in comments:
text = comment.body
author = comment.author
if 'CHOOSE_A_KEYWORD_HERE' in text.lower():
CHOSEN_REPONSE = random.choice(AVAILABLE_RESPONSES)
message = CHOSEN_REPONSE.format(author)
comment.reply(message)
time.sleep(600)
This second script will browse r/All endlessly, and upon encountering a designated KEYWORD, it will submit a comment response taken randomly from an array of pre-defined strings, before sleeping for 10 minutes.
This could be used to distribute lovely compliments in response to polite comments...or for other, less wholesome purposes. Whatever the purpose, be sure to adhere to Reddit's API and site-wide content rules, and to not abuse the power of anonymity.
Speaking of which, here is an example Bot script:
#!/usr/bin/python
import praw
import time
import random
import markovify
reddit = praw.Reddit('MY_BOT_USERNAME')
subreddit = reddit.subreddit("All")
comments = subreddit.stream.comments()
signalsentence="These are words to look for :)"
signals = signalsentence.split()
keyword=random.choice(signals)
print keyword
chattingshit = [
]
# Get raw text as string.
with open("/path/to/corpus.txt") as f:
textsource = f.read()
# Build the model.
text_model = markovify.NewlineText(textsource)
try:
for comment in comments:
author = comment.author # Fetch author
text = comment.body # Fetch body
if keyword in text.lower():
if author != "MY_BOT_USERNAME":
# Generate a message
for i in range(3):
shaekeitUp = text_model.make_sentence()
chattingshit.append(shaekeitUp)
print shaekeitUp
upboats = comment.score
print upboats
signalsentence=random.choice(text.split())
postingshit = random.choice(chattingshit)
message = postingshit.format(author)
comment.reply(message) # Send message
chattingshit.append(text)
print message
time.sleep(20)
for submission in subreddit.hot(limit=10):
print("Title: ", submission.title)
print("Text: ", submission.selftext)
print("Score: ", submission.score)
print("---------------------------------\n")
except KeyboardInterrupt:
print("Shutting down.")
quit()
except praw.errors.HTTPException as e:
exc = e._raw
print("Some thing bad happened! HTTPError", exc.status_code)
if exc.status_code == 503:
print("Let's wait til reddit comes back! Sleeping 60 seconds.")
time.sleep(60)
except Exception as e:
print("Some thing bad happened! :O ", e)
traceback.print_exc()
This was a quick look at building bots in Python, and I hope to revisit this in the future with some more advanced concepts.